Name | Description | In Design Patterns | In Code Complete[14] | Other |
---|---|---|---|---|
Abstract factory | Provide an interface for creating families of related or dependent objects without specifying their concrete classes. | Yes | Yes | OK |
Builder | Separate the construction of a complex object from its representation, allowing the same construction process to create various representations. | Yes | No | OK |
Dependency Injection | A class accepts the objects it requires from an injector instead of creating the objects directly. | No | No | OK |
Factory method | Define an interface for creating a single object, but let subclasses decide which class to instantiate. Factory Method lets a class defer instantiation to subclasses. | Yes | Yes | OK |
Lazy initialization | Tactic of delaying the creation of an object, the calculation of a value, or some other expensive process until the first time it is needed. This pattern appears in the GoF catalog as "virtual proxy", an implementation strategy for the Proxy pattern. | No | No | PoEAA[15] |
Multiton | Ensure a class has only named instances, and provide a global point of access to them. | No | No | — |
Object pool | Avoid expensive acquisition and release of resources by recycling objects that are no longer in use. Can be considered a generalisation of connection pool and thread pool patterns. | No | No | — |
Prototype | Specify the kinds of objects to create using a prototypical instance, and create new objects from the 'skeleton' of an existing object, thus boosting performance and keeping memory footprints to a minimum. | Yes | No | OK |
Resource acquisition is initialization (RAII) | Ensure that resources are properly released by tying them to the lifespan of suitable objects. | No | No | — |
Singleton | Ensure a class has only one instance, and provide a global point of access to it. | Yes | Yes | OK |
Adapter, Wrapper, or Translator | Convert the interface of a class into another interface clients expect. An adapter lets classes work together that could not otherwise because of incompatible interfaces. The enterprise integration pattern equivalent is the translator. | Yes | Yes | — |
Bridge | Decouple an abstraction from its implementation allowing the two to vary independently. | Yes | Yes | — |
Composite | Compose objects into tree structures to represent part-whole hierarchies. Composite lets clients treat individual objects and compositions of objects uniformly. | Yes | Yes | — |
Decorator | Attach additional responsibilities to an object dynamically keeping the same interface. Decorators provide a flexible alternative to subclassing for extending functionality. | Yes | Yes | — |
Delegation | Extent a class by composition instead of subclassing. The object handles a request by delegating to a second object (the delegate) | — | — | — |
Extension object | Adding functionality to a hierarchy without changing the hierarchy. | No | No | Agile Software Development, Principles, Patterns, and Practices[16] |
Facade | Provide a unified interface to a set of interfaces in a subsystem. Facade defines a higher-level interface that makes the subsystem easier to use. | Yes | Yes | — |
Flyweight | Use sharing to support large numbers of similar objects efficiently. | Yes | No | — |
Front controller | The pattern relates to the design of Web applications. It provides a centralized entry point for handling requests. | No | No | |
Marker | Empty interface to associate metadata with a class. | No | No | Effective Java[19] |
Module | Group several related elements, such as classes, singletons, methods, globally used, into a single conceptual entity. | No | No | — |
Proxy | Provide a surrogate or placeholder for another object to control access to it. | Yes | No | — |
Twin[20] | Twin allows modeling of multiple inheritance in programming languages that do not support this feature. | No | No | — |
Blackboard | Artificial intelligence pattern for combining disparate sources of data (see blackboard system) | No | No | — |
Chain of responsibility | Avoid coupling the sender of a request to its receiver by giving more than one object a chance to handle the request. Chain the receiving objects and pass the request along the chain until an object handles it. | Yes | No | OK (DEV) |
Command | Encapsulate a request as an object, thereby allowing for the parameterization of clients with different requests, and the queuing or logging of requests. It also allows for the support of undoable operations. | Yes | No | OK |
Interpreter | Given a language, define a representation for its grammar along with an interpreter that uses the representation to interpret sentences in the language. | Yes | No | — |
Iterator | Provide a way to access the elements of an aggregate object sequentially without exposing its underlying representation. | Yes | Yes | — |
Mediator | Define an object that encapsulates how a set of objects interact. Mediator promotes loose coupling by keeping objects from referring to each other explicitly, and it allows their interaction to vary independently. | Yes | No | — |
Memento | Without violating encapsulation, capture and externalize an object's internal state allowing the object to be restored to this state later. | Yes | No | — |
Null object | Avoid null references by providing a default object. | No | No | — |
Observer or Publish/subscribe | Define a one-to-many dependency between objects where a state change in one object results in all its dependents being notified and updated automatically. | Yes | Yes | — |
Servant | Define common functionality for a group of classes. The servant pattern is also frequently called helper class or utility class implementation for a given set of classes. The helper classes generally have no objects hence they have all static methods that act upon different kinds of class objects. | No | No | — |
Specification | Recombinable business logic in a Boolean fashion. | No | No | — |
State | Allow an object to alter its behavior when its internal state changes. The object will appear to change its class. | Yes | No | — |
Strategy | Define a family of algorithms, encapsulate each one, and make them interchangeable. Strategy lets the algorithm vary independently from clients that use it. | Yes | Yes | — |
Template method | Define the skeleton of an algorithm in an operation, deferring some steps to subclasses. Template method lets subclasses redefine certain steps of an algorithm without changing the algorithm's structure. | Yes | Yes | — |
Visitor | Represent an operation to be performed on instances of a set of classes. Visitor lets a new operation be defined without changing the classes of the elements on which it operates. | Yes | No | — |
Fluent Interface | Design an API to be method chained so that it reads like a DSL. Each method call returns a context through which the next logical method call(s) are made available. | No | No | — |
Active Object | Decouples method execution from method invocation that reside in their own thread of control. The goal is to introduce concurrency, by using asynchronous method invocation and a scheduler for handling requests. | Yes | — | |
Balking | Only execute an action on an object when the object is in a particular state. | No | — | |
Binding properties | Combining multiple observers to force properties in different objects to be synchronized or coordinated in some way.[22] | No | — | |
Compute kernel | The same calculation many times in parallel, differing by integer parameters used with non-branching pointer math into shared arrays, such as GPU-optimized Matrix multiplication or Convolutional neural network. | No | — | |
Double-checked locking | Reduce the overhead of acquiring a lock by first testing the locking criterion (the 'lock hint') in an unsafe manner; only if that succeeds does the actual locking logic proceed. Can be unsafe when implemented in some language/hardware combinations. It can therefore sometimes be considered an anti-pattern. | Yes | — | |
Event-based asynchronous | Addresses problems with the asynchronous pattern that occur in multithreaded programs.[23] | No | — | |
Guarded suspension | Manages operations that require both a lock to be acquired and a precondition to be satisfied before the operation can be executed. | No | — | |
Join | Join-pattern provides a way to write concurrent, parallel and distributed programs by message passing. Compared to the use of threads and locks, this is a high-level programming model. | No | — | |
Lock | One thread puts a "lock" on a resource, preventing other threads from accessing or modifying it.[24] | No | PoEAA[15] | |
Messaging design pattern (MDP) | Allows the interchange of information (i.e. messages) between components and applications. | No | — | |
Monitor object | An object whose methods are subject to mutual exclusion, thus preventing multiple objects from erroneously trying to use it at the same time. | Yes | — | |
Reactor | A reactor object provides an asynchronous interface to resources that must be handled synchronously. | Yes | — | |
Read-write lock | Allows concurrent read access to an object, but requires exclusive access for write operations. An underlying semaphore might be used for writing, and a Copy-on-write mechanism may or may not be used. | No | — | |
Scheduler | Explicitly control when threads may execute single-threaded code. | No | — | |
Thread pool | A number of threads are created to perform a number of tasks, which are usually organized in a queue. Typically, there are many more tasks than threads. Can be considered a special case of the object pool pattern. | No | — | |
Thread-specific storage | Static or "global" memory local to a thread. | Yes | — | |
Safe Concurrency with Exclusive Ownership | Avoiding the need for runtime concurrent mechanisms, because exclusive ownership can be proven. This is a notable capability of the Rust language, but compile-time checking isn't the only means, a programmer will often manually design such patterns into code - omitting the use of locking mechanism because the programmer assesses that a given variable is never going to be concurrently accessed. | No | — | |
CPU atomic operation | x86 and other CPU architectures support a range of atomic instructions that guarantee memory safety for modifying and accessing primitive values (integers). For example, two threads may both increment a counter safely. These capabilities can also be used to implement the mechanisms for other concurrency patterns as above. The C# language uses the Interlocked class for these capabilities. | No | — | |
Choreography | Have each component of the system participate in the decision-making process about the workflow of a business transaction, instead of relying on a central point of control. | Yes | OK https://learn.microsoft.com/en-us/azure/ar chitecture/patterns/choreography | |
Strangler Fig Facade | Incrementally migrate a legacy system by gradually replacing specific pieces of functionality with new applications and services. As features from the legacy system are replaced, the new system eventually replaces all of the old system's features, strangling the old system and allowing you to decommission it. | Yes | OK https://learn.microsoft.com/en-us/azure/architecture/patterns/_images/strangler.png |
SA
Saturday 4 February 2023
Design Patterns
Wednesday 21 February 2018
Spring MVC
1. What is Spring Framework?
Spring is the most broadly used framework for the development of Java Enterprise Edition applications. The core features of Spring can be used in developing any Java application.
We can use its extensions for building various web applications on top of the Java EE platform, or we may just use its dependency injection provisions in simple standalone applications.
2. What are the benefits of using Spring?
Spring targets to make Java EE development easier. Here are the advantages of using it:
- Lightweight: there is a slight overhead of using the framework in development
- Inversion of Control (IoC): Spring container takes care of wiring dependencies of various objects, instead of creating or looking for dependent objects
- Aspect Oriented Programming (AOP): Spring supports AOP to separate business logic from system services
- IoC container: it manages Spring Bean life cycle and project specific configurations
- MVC framework: that is used to create web applications or RESTful web services, capable of returning XML/JSON responses
- Transaction management: reduces the amount of boiler-plate code in JDBC operations, file uploading, etc., either by using Java annotations or by Spring Bean XML configuration file
- Exception Handling: Spring provides a convenient API for translating technology-specific exceptions into unchecked exceptions
3. What Spring sub-projects do you know? Describe them briefly.
- Core – a key module that provides fundamental parts of the framework, like IoC or DI
- JDBC – this module enables a JDBC-abstraction layer that removes the need to do JDBC coding for specific vendor databases
- ORM integration – provides integration layers for popular object-relational mapping APIs, such as JPA, JDO, and Hibernate
- Web – a web-oriented integration module, providing multipart file upload, Servlet listeners, and web-oriented application context functionalities
- MVC framework – a web module implementing the Model View Controller design pattern
- AOP module – aspect-oriented programming implementation allowing the definition of clean method-interceptors and pointcuts
4. What is Dependency Injection?
Dependency Injection, an aspect of Inversion of Control (IoC), is a general concept stating that you do not create your objects manually but instead describe how they should be created. An IoC container will instantiate required classes if needed.
For more details, please refer here.
5. How can we inject beans in Spring?
A few different options exist:
- Setter Injection
- Constructor Injection
- Field Injection
The configuration can be done using XML files or annotations.
For more details, check this article.
6. Which is the best way of injecting beans and why?
The recommended approach is to use constructor arguments for mandatory dependencies and setters for optional ones. Constructor injection allows injecting values to immutable fields and makes testing easier.
7. What is the difference between BeanFactory and ApplicationContext?
BeanFactory is an interface representing a container that provides and manages bean instances. The default implementation instantiates beans lazily when getBean() is called.
ApplicationContext is an interface representing a container holding all information, metadata, and beans in the application. It also extends the BeanFactory interface but the default implementation instantiates beans eagerly when the application starts. This behavior can be overridden for individual beans.
For all differences, please refer to the reference.
8. What is a Spring Bean?
The Spring Beans are Java Objects that are initialized by the Spring IoC container.
9. What is the default bean scope in Spring framework?
By default, a Spring Bean is initialized as a singleton.
10. How to define the scope of a bean?
To set Spring Bean’s scope, we can use @Scope annotation or “scope” attribute in XML configuration files. There are five supported scopes:
- singleton
- prototype
- request
- session
- global-session
For differences, please refer here.
11. Are singleton beans thread-safe?
No, singleton beans are not thread-safe, as thread safety is about execution, whereas the singleton is a design pattern focusing on creation. Thread safety depends only on the bean implementation itself.
12. What does the Spring bean lifecycle look like?
First, a Spring bean needs to be instantiated, based on Java or XML bean definition. It may also be required to perform some initialization to get it into a usable state. After that, when the bean is no longer required, it will be removed from the IoC container.
The whole cycle with all initialization methods is shown on the image (source):
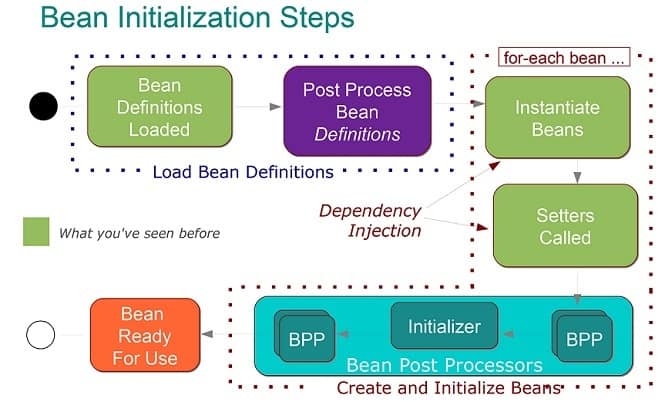
13. What is the Spring Java-Based Configuration?
It’s one of the ways of configuring Spring-based applications in a type-safe manner. It’s an alternative to the XML-based configuration.
Also, if you want to migrate your project from XML to Java config, please refer to this article.
14. Can we have multiple Spring configuration files in one project?
Yes, in large projects, having multiple Spring configurations is recommended to increase maintainability and modularity.
You can load multiple Java-based configuration files:
1
2
3
| @Configuration @Import ({MainConfig. class , SchedulerConfig. class }) public class AppConfig { |
Or load one XML file that will contain all other configs:
1
| ApplicationContext context = new ClassPathXmlApplicationContext( "spring-all.xml" ); |
And inside this XML file you’ll have:
1
2
| < import resource = "main.xml" /> < import resource = "scheduler.xml" /> |
15. What is Spring Security?
Spring Security is a separate module of the Spring framework that focuses on providing authentication and authorization methods in Java applications. It also takes care of most of the common security vulnerabilities such as CSRF attacks.
To use Spring Security in web applications, you can get started with a simple annotation: @EnableWebSecurity.
You can find the whole series of articles related to security on Baeldung.
16. What is Spring Boot?
Spring Boot is a project that provides a pre-configured set of frameworks to reduce boilerplate configuration so that you can have a Spring application up and running with the smallest amount of code.
17. Name some of the Design Patterns used in the Spring Framework?
- Singleton Pattern: Singleton-scoped beans
- Factory Pattern: Bean Factory classes
- Prototype Pattern: Prototype-scoped beans
- Adapter Pattern: Spring Web and Spring MVC
- Proxy Pattern: Spring Aspect Oriented Programming support
- Template Method Pattern: JdbcTemplate, HibernateTemplate, etc.
- Front Controller: Spring MVC DispatcherServlet
- Data Access Object: Spring DAO support
- Model View Controller: Spring MVC
18. How does the scope Prototype work?
Scope prototype means that every time you call for an instance of the Bean, Spring will create a new instance and return it. This differs from the default singleton scope, where a single object instance is instantiated once per Spring IoC container.
3. Spring MVC
19. How to Get ServletContext and ServletConfig Objects in a Spring Bean?
You can do either by:
- Implementing Spring-aware interfaces. The complete list is available here.
- Using @Autowired annotation on those beans:
1
2
3
4
5
| @Autowired ServletContext servletContext; @Autowired ServletConfig servletConfig; |
20. What is the role of the @Required annotation?
The @Required annotation is used on setter methods, and it indicates that the bean property that has this annotation must be populated at configuration time. Otherwise, the Spring container will throw a BeanInitializationException exception.
Also, @Required differs from @Autowired – as it is limited to a setter, whereas @Autowired is not. @Autowired can be used to wire with a constructor and a field as well, while @Required only checks if the property is set.
Let’s see an example:
1
2
3
4
5
6
7
8
| public class Person { private String name; @Required public void setName(String name) { this .name = name; } } |
Now, the name of the Person bean needs to be set in XML config like this:
1
2
3
| < bean id = "person" class = "com.baeldung.Person" > < property name = "name" value = "Joe" /> </ bean > |
Please note that @Required doesn’t work with Java based @Configuration classes by default. If you need to make sure that all your properties are set, you can do so when you create the bean in the @Bean annotated methods.
21. What is the role of the @Autowired annotation?
The @Autowired annotation can be used with fields or methods for injecting a bean by type. This annotation allows Spring to resolve and inject collaborating beans into your bean.
For more details, please refer to this tutorial.
22. What is the Role of the @Qualifier Annotation?
It is used simultaneously with the @Autowired annotation to avoid confusion when multiple instances of a bean type are present.
Let’s see an example. We declared two similar beans in XML config:
1
2
3
4
5
6
| < bean id = "person1" class = "com.baeldung.Person" > < property name = "name" value = "Joe" /> </ bean > < bean id = "person2" class = "com.baeldung.Person" > < property name = "name" value = "Doe" /> </ bean > |
When we try to wire the bean, we’ll get an org.springframework.beans.factory.NoSuchBeanDefinitionException. To fix it, we need to use @Qualifier to tell Spring about which bean should be wired:
1
2
3
| @Autowired @Qualifier ( "person1" ) private Person person; |
23. How to handle exceptions in Spring MVC environment?
There are three ways to handle exceptions in Spring MVC:
- Using @ExceptionHandler at controller level – this approach has a major feature – the @ExceptionHandler annotated method is only active for that particular controller, not globally for the entire application
- Using HandlerExceptionResolver – this will resolve any exception thrown by the application
- Using @ControllerAdvice – Spring 3.2 brings support for a global @ExceptionHandler with the @ControllerAdvice annotation, which enables a mechanism that breaks away from the older MVC model and makes use of ResponseEntity along with the type safety and flexibility of @ExceptionHandler
For more detailed information on this topic, go through this writeup.
24. How to validate if the bean was initialized using valid values?
Spring supports JSR-303 annotation-based validations. JSR-303 is a specification of the Java API for bean validation, part of JavaEE and JavaSE, which ensures that properties of a bean meet specific criteria, using annotations such as @NotNull, @Min, and @Max. The article regarding JSR-303 is available here.
What’s more, Spring provides the Validator interface for creating custom validators. For example, you can have a look here.
25. What is Spring MVC Interceptor and how to use it?
Spring MVC Interceptors allow us to intercept a client request and process it at three places – before handling, after handling, or after completion (when the view is rendered) of a request.
The interceptor can be used for cross-cutting concerns and to avoid repetitive handler code like logging, changing globally used parameters in Spring model, etc.
For details and various implementations, take a look at this series.
26. What is a Controller in Spring MVC?
Simply put, all the requests processed by the DispatcherServlet are directed to classes annotated with @Controller. Each controller class maps one or more requests to methods that process and execute the requests with provided inputs.
If you need to take a step back, we recommend having a look at the concept of the Front Controller in the typical Spring MVC architecture.
4. Spring Web
27. How does the @RequestMapping annotation work?
The @RequestMapping annotation is used to map web requests to Spring Controller methods. In addition to simple use cases, we can use it for mapping of HTTP headers, binding parts of the URI with @PathVariable, and working with URI parameters and the @RequestParam annotation.
More details on @RequestMapping are available here.
28. What’s the Difference Between @Controller, @Component, @Repository, and @Service Annotations in Spring?
According to the official Spring documentation, @Component is a generic stereotype for any Spring-managed component. @Repository, @Service, and @Controller are specializations of @Component for more specific use cases, for example, in the persistence, service, and presentation layers, respectively.
Let’s take a look at specific use cases of last three:
- @Controller – indicates that the class serves the role of a controller, and detects @RequestMappingannotations within the class
- @Service – indicates that the class holds business logic and calls methods in the repository layer
- @Repository – indicates that the class defines a data repository; its job is to catch platform-specific exceptions and re-throw them as one of Spring’s unified unchecked exceptions
29. What are DispatcherServlet and ContextLoaderListener?
Simply put, in the Front Controller design pattern, a single controller is responsible for directing incoming HttpRequests to all of an application’s other controllers and handlers.
Spring’s DispatcherServlet implements this pattern and is, therefore, responsible for correctly coordinating the HttpRequests to the right handlers.
On the other hand, ContextLoaderListener starts up and shuts down Spring’s root WebApplicationContext. It ties the lifecycle of ApplicationContext to the lifecycle of the ServletContext. We can use it to define shared beans working across different Spring contexts.
For more details on DispatcherServler, please refer to this tutorial.
30. What is ViewResolver in Spring?
The ViewResolver enables an application to render models in the browser – without tying the implementation to a specific view technology – by mapping view names to actual views.
For a guide to the ViewResolver, have a look here.
31. What is a MultipartResolver and when is it used?
The MultipartResolver interface is used for uploading files. The Spring framework provides one MultipartResolver implementation for use with Commons FileUpload and another for use with Servlet 3.0 multipart request parsing.
Using these, we can support file uploads in our web applications.
5. Spring Data Access
32. What is Spring JDBCTemplate class and how to use it?
The Spring JDBC template is the primary API through which we can access database operations logic that we’re interested in:
- creation and closing of connections
- executing statements and stored procedure calls
- iterating over the ResultSet and returning results
To use it, we’ll need to define the simple configuration of DataSource:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
| @Configuration @ComponentScan ( "org.baeldung.jdbc" ) public class SpringJdbcConfig { @Bean public DataSource mysqlDataSource() { DriverManagerDataSource dataSource = new DriverManagerDataSource(); dataSource.setDriverClassName( "com.mysql.jdbc.Driver" ); dataSource.setUsername( "guest_user" ); dataSource.setPassword( "guest_password" ); return dataSource; } } |
For further explanation, you can go through this quick article.
33. How would you enable transactions in Spring and what are their benefits?
There are two distinct ways to configure Transactions – with annotations or by using Aspect Oriented Programming (AOP) – each with their advantages.
The benefits of using Spring Transactions, according to the official docs, are:
- Provide a consistent programming model across different transaction APIs such as JTA, JDBC, Hibernate, JPA, and JDO
- Support declarative transaction management
- Provide a simpler API for programmatic transaction management than some complex transaction APIs such as JTA
- Integrate very well with Spring’s various data access abstractions
34. What is Spring DAO?
Spring Data Access Object is Spring’s support provided to work with data access technologies like JDBC, Hibernate, and JPA in a consistent and easy way.
You can, of course, go more in-depth on persistence, with the entire series discussing persistence in Spring.
6. Spring Aspect-Oriented Programming (AOP)
35. What is Aspect-Oriented Programming?
Aspects enable the modularization of cross-cutting concerns such as transaction management that span multiple types and objects by adding extra behavior to already existing code without modifying affected classes.
Here is the example of aspect-based execution time logging.
36. What are Aspect, Advice, Pointcut, and JoinPoint in AOP?
- Aspect: a class that implements cross-cutting concerns, such as transaction management
- Advice: the methods that get executed when a specific JoinPoint with matching Pointcut is reached in the application
- Pointcut: a set of regular expressions that are matched with JoinPoint to determine whether Adviceneeds to be executed or not
- JoinPoint: a point during the execution of a program, such as the execution of a method or the handling of an exception
37. What is Weaving?
According to the official docs, weaving is a process that links aspects with other application types or objects to create an advised object. This can be done at compile time, load time, or at runtime. Spring AOP, like other pure Java AOP frameworks, performs weaving at runtime.
Subscribe to:
Posts (Atom)